OAuth2.0
We use the OAuth2.0 standard as authentication flow.
Therefore, to use our API, you will need to provide the client credentials obtained through our Partner Registration.
If you do not have these credentials please contact our team via email tech.services@a55.tech.
Flowchart:
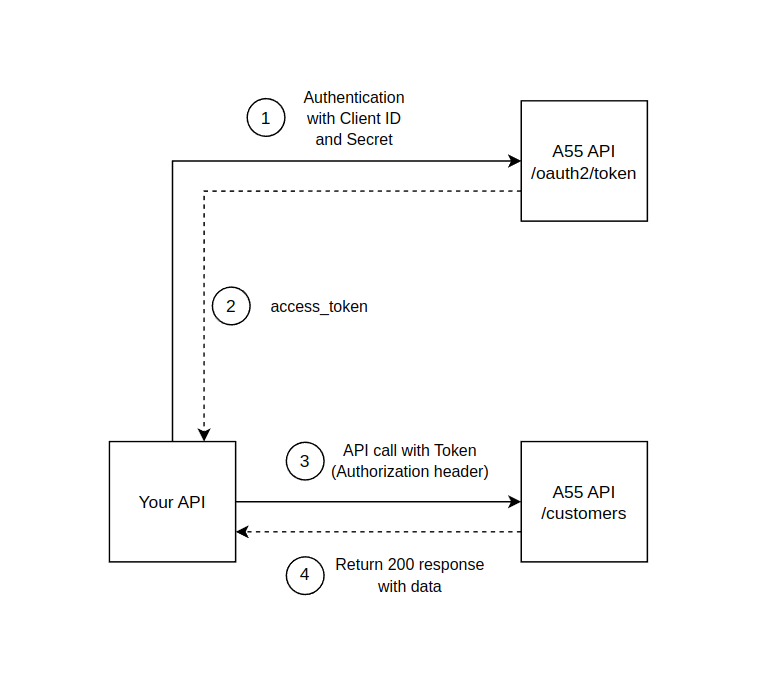
DO NOT SHARE YOUR CLIENT SECRET
Your CLIENT_SECRET must not be shared with third parties or used in frontend applications.
Here is an example of how to request the token in Node JS:
import axios from 'axios';
const options = {
method: 'POST',
url: '{URL}/api/v1/oauth2/token',
params: {
grant_type: 'client_credentials',
client_id: '<YOUR_CLIENT_ID>',
client_secret: '<YOUR_CLIENT_SECRET>'
},
headers: {
'Content-Type': 'application/x-www-form-urlencoded',
},
};
axios
.request(options)
.then(function (response) {
console.log(response.data);
// {
// "access_token": "<token>",
// "expires_in": 3600,
// "token_type": "Bearer"
// }
})
.catch(function (error) {
console.error(error);
});
After obtaining the "access_token", simply send it in the Authorization
header in subsequent requests:
import axios from 'axios';
const options = {
method: 'POST',
url: '{URL}/api/v1/bank/wallet',
headers: {
Authorization: 'Bearer <access_token>', // aqui
'Content-Type': 'application/json',
},
data: { ... }
};
axios
.request(options)
.then(function (response) {
console.log(response.data);
})
.catch(function (error) {
console.error(error);
});
Updated 7 months ago